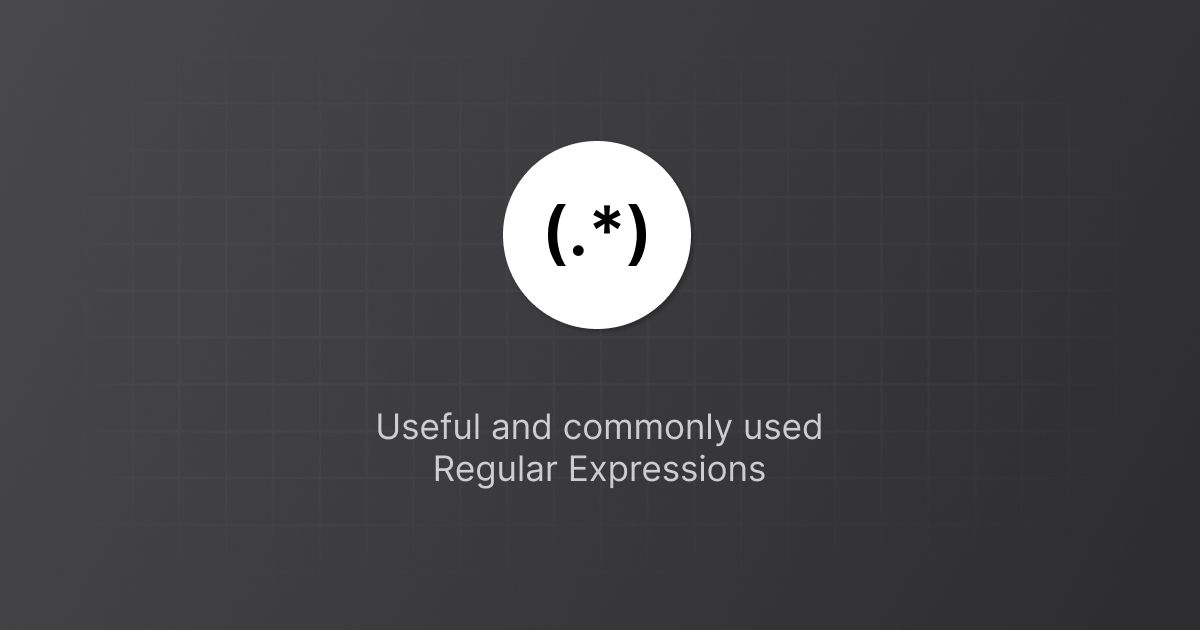
Regular Expressions (Regex) are expressions that define a search pattern. Regex can be implemented in various tasks including searching, replacing, validation and text processing/parsing. It is mostly known in the combination with the programming language Perl, but Regex are also supported by most other languages.
If you every want to really dive deep into the world of Regular Expressions, I'd recommend the book "Mastering Regular Expressions " by Jeffrey E.F. Friedl (Amazon US, Amazon DE | no affiliate).
Testing your regular expressions
There are times in which you can't test your Regex while programming. Or you might just want to play around and test your Regex. In any case, there are a few Regex tester online, where you can add sample data and build or test your regex. Some of them are:
Commonly used
Digits
Whole numbers:
/^\d+$/
Decimal numbers:
/^\d*\.\d+$/
Whole and decimal numbers:
/^\d*(\.\d+)?$/
Negative or positive whole and decimal numbers:
/^-?\d*(\.\d+)?$/
Alphanumeric
Without space
/^[a-zA-Z0-9]*$/
With Space
/^[a-zA-Z0-9 ]*$/
Username
Alphanumeric lowercase username that might contain
- numbers
- '-' or '_'
and a length of 3-16 characters
/^[a-z0-9_-]{3,16}$/
Common email
/^([a-zA-Z0-9._%-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,6})*$/
Uncommon email
e.g. [email protected]
/^([a-z0-9_\.\+-]+)@([\da-z\.-]+)\.([a-z\.]{2,6})$/
RFC 5322
/(?(DEFINE)
(?<addr_spec> (?&local_part) @ (?&domain) )
(?<local_part> (?&dot_atom) | (?"ed_string) | (?&obs_local_part) )
(?<domain> (?&dot_atom) | (?&domain_literal) | (?&obs_domain) )
(?<domain_literal> (?&CFWS)? \[ (?: (?&FWS)? (?&dtext) )* (?&FWS)? \] (?&CFWS)? )
(?<dtext> [\x21-\x5a] | [\x5e-\x7e] | (?&obs_dtext) )
(?<quoted_pair> \\ (?: (?&VCHAR) | (?&WSP) ) | (?&obs_qp) )
(?<dot_atom> (?&CFWS)? (?&dot_atom_text) (?&CFWS)? )
(?<dot_atom_text> (?&atext) (?: \. (?&atext) )* )
(?<atext> [a-zA-Z0-9!#$%&'*+\/=?^_`{|}~-]+ )
(?<atom> (?&CFWS)? (?&atext) (?&CFWS)? )
(?<word> (?&atom) | (?"ed_string) )
(?<quoted_string> (?&CFWS)? " (?: (?&FWS)? (?&qcontent) )* (?&FWS)? " (?&CFWS)? )
(?<qcontent> (?&qtext) | (?"ed_pair) )
(?<qtext> \x21 | [\x23-\x5b] | [\x5d-\x7e] | (?&obs_qtext) )
# comments and whitespace
(?<FWS> (?: (?&WSP)* \r\n )? (?&WSP)+ | (?&obs_FWS) )
(?<CFWS> (?: (?&FWS)? (?&comment) )+ (?&FWS)? | (?&FWS) )
(?<comment> \( (?: (?&FWS)? (?&ccontent) )* (?&FWS)? \) )
(?<ccontent> (?&ctext) | (?"ed_pair) | (?&comment) )
(?<ctext> [\x21-\x27] | [\x2a-\x5b] | [\x5d-\x7e] | (?&obs_ctext) )
# obsolete tokens
(?<obs_domain> (?&atom) (?: \. (?&atom) )* )
(?<obs_local_part> (?&word) (?: \. (?&word) )* )
(?<obs_dtext> (?&obs_NO_WS_CTL) | (?"ed_pair) )
(?<obs_qp> \\ (?: \x00 | (?&obs_NO_WS_CTL) | \n | \r ) )
(?<obs_FWS> (?&WSP)+ (?: \r\n (?&WSP)+ )* )
(?<obs_ctext> (?&obs_NO_WS_CTL) )
(?<obs_qtext> (?&obs_NO_WS_CTL) )
(?<obs_NO_WS_CTL> [\x01-\x08] | \x0b | \x0c | [\x0e-\x1f] | \x7f )
# character class definitions
(?<VCHAR> [\x21-\x7E] )
(?<WSP> [ \t] )
)
^(?&addr_spec)$/xmg
Passwords
Moderate Password
Password with at least
- 1 lowercase letter
- 1 uppercase letter
- 1 number
and a minimum length of 8 characters.
Spaces allowed.
/(?=(.*[0-9]))((?=.*[A-Za-z0-9])(?=.*[A-Z])(?=.*[a-z]))^.{8,}$/
Spaces not allowed.
/^((?=\S*?[A-Z])(?=\S*?[a-z])(?=\S*?[0-9]).{8,})\S$/
With optional special character and spaces not allowed
/^(?=.*\d)(?=.*[a-zA-Z])[0-9a-zA-Z$&+,:;=?@#|'<>.-^*()%!]{8,}$/
Strong Password
Passwords with at least
- 1 lowercase letter
- 1 uppercase letter
- 1 number
- 1 special character
and a minimum of 8 characters with spaces not allowed.
All special characters
/^(?=.*\d)(?=.*[A-Z])(?=.*[a-z])(?=.*[^\w\d\s:])([^\s]){8,}$/
One of these special characters: !@#$%^&*()-=¡£_+
~.,<>/?;:'"|[]{}`
^(?=.*\d)(?=.*[A-Z])(?=.*[a-z])(?=.*[\!\@\#\$\%\^\&\*\(\)\-\=\¡\£\_\+\`\~\.\,\<\>\/\?\;\:\'\"\\\|\[\]\{\}])([^\s]){8,}$
Strong+ Password
Password with minimum
- 1 lowercase letter
- 1 uppercase letter
- 1 number
- 1 special character
- 12 characters
and does not contain certain words.
^(?=.*\d)(?=.*[a-z])(?=.*[A-Z])(?=.*[^\w\s\d:])(?=(?:([\w\d])\1?(?!\1\1)))(?!(?=.*([Jj]ohn|[Dd]oe]|blabla))).{12,}$
IP addresses
IPv4
/^(?:(?:25[0-5]|2[0-4]\d|1?\d?\d)(?:\.(?!$)|$)){4}$/
Private IPv4
/^(10(\.(1?\d\d?|2([0-4]\d?|5[0-5])))(?2)|172\.(1[6-9]|2\d|3[0-2])(?2)|192\.168(?2))(?2)$/
IPv6
/(?:(?:(?:[A-F0-9]{1,4}:){6}|(?=(?:[A-F0-9]{0,4}:){0,6}(?:[0-9]{1,3}\.){3}[0-9]{1,3}(?![:.\w]))(([0-9A-F]{1,4}:){0,5}|:)((:[0-9A-F]{1,4}){1,5}:|:)|::(?:[A-F0-9]{1,4}:){5})(?:(?:25[0-5]|2[0-4][0-9]|1[0-9][0-9]|[1-9]?[0-9])\.){3}(?:25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)|(?:[A-F0-9]{1,4}:){7}[A-F0-9]{1,4}|(?=(?:[A-F0-9]{0,4}:){0,7}[A-F0-9]{0,4}(?![:.\w]))(([0-9A-F]{1,4}:){1,7}|:)((:[0-9A-F]{1,4}){1,7}|:)|(?:[A-F0-9]{1,4}:){7}:|:(:[A-F0-9]{1,4}){7})(?![:.\w])/i
With Subnet
/(?:(?:(?:[A-F0-9]{1,4}:){6}|(?=(?:[A-F0-9]{0,4}:){0,6}(?:[0-9]{1,3}\.){3}[0-9]{1,3}(?![:.\w]))(([0-9A-F]{1,4}:){0,5}|:)((:[0-9A-F]{1,4}){1,5}:|:)|::(?:[A-F0-9]{1,4}:){5})(?:(?:25[0-5]|2[0-4][0-9]|1[0-9][0-9]|[1-9]?[0-9])\.){3}(?:25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)|(?:[A-F0-9]{1,4}:){7}[A-F0-9]{1,4}|(?=(?:[A-F0-9]{0,4}:){0,7}[A-F0-9]{0,4}(?![:.\w]))(([0-9A-F]{1,4}:){1,7}|:)((:[0-9A-F]{1,4}){1,7}|:)|(?:[A-F0-9]{1,4}:){7}:|:(:[A-F0-9]{1,4}){7})(?![:.\w])\/(?:12[0-8]|1[01][0-9]|[1-9]?[0-9])/i
IPv4 or IPv6
/((^\s*((([0-9]|[1-9][0-9]|1[0-9]{2}|2[0-4][0-9]|25[0-5])\.){3}([0-9]|[1-9][0-9]|1[0-9]{2}|2[0-4][0-9]|25[0-5]))\s*$)|(^\s*((([0-9A-Fa-f]{1,4}:){7}([0-9A-Fa-f]{1,4}|:))|(([0-9A-Fa-f]{1,4}:){6}(:[0-9A-Fa-f]{1,4}|((25[0-5]|2[0-4]\d|1\d\d|[1-9]?\d)(\.(25[0-5]|2[0-4]\d|1\d\d|[1-9]?\d)){3})|:))|(([0-9A-Fa-f]{1,4}:){5}(((:[0-9A-Fa-f]{1,4}){1,2})|:((25[0-5]|2[0-4]\d|1\d\d|[1-9]?\d)(\.(25[0-5]|2[0-4]\d|1\d\d|[1-9]?\d)){3})|:))|(([0-9A-Fa-f]{1,4}:){4}(((:[0-9A-Fa-f]{1,4}){1,3})|((:[0-9A-Fa-f]{1,4})?:((25[0-5]|2[0-4]\d|1\d\d|[1-9]?\d)(\.(25[0-5]|2[0-4]\d|1\d\d|[1-9]?\d)){3}))|:))|(([0-9A-Fa-f]{1,4}:){3}(((:[0-9A-Fa-f]{1,4}){1,4})|((:[0-9A-Fa-f]{1,4}){0,2}:((25[0-5]|2[0-4]\d|1\d\d|[1-9]?\d)(\.(25[0-5]|2[0-4]\d|1\d\d|[1-9]?\d)){3}))|:))|(([0-9A-Fa-f]{1,4}:){2}(((:[0-9A-Fa-f]{1,4}){1,5})|((:[0-9A-Fa-f]{1,4}){0,3}:((25[0-5]|2[0-4]\d|1\d\d|[1-9]?\d)(\.(25[0-5]|2[0-4]\d|1\d\d|[1-9]?\d)){3}))|:))|(([0-9A-Fa-f]{1,4}:){1}(((:[0-9A-Fa-f]{1,4}){1,6})|((:[0-9A-Fa-f]{1,4}){0,4}:((25[0-5]|2[0-4]\d|1\d\d|[1-9]?\d)(\.(25[0-5]|2[0-4]\d|1\d\d|[1-9]?\d)){3}))|:))|(:(((:[0-9A-Fa-f]{1,4}){1,7})|((:[0-9A-Fa-f]{1,4}){0,5}:((25[0-5]|2[0-4]\d|1\d\d|[1-9]?\d)(\.(25[0-5]|2[0-4]\d|1\d\d|[1-9]?\d)){3}))|:)))(%.+)?\s*$))/
Domains & URLs
General Domain (up to 3rd-level-domain)
/(?:(?:([\w\-]*)(?:\.))?([\w\-]*))\.(\w*)/
With optional port:
/(?:(?:([\w\-]*)(?:\.))?([\w\-]*))\.(\w*)(?:\:(\d*))?/
With protocol and optional port:
/(\w*)\:\/\/(?:(?:([\w\-]*)(?:\.))?([\w\-]*))\.(\w*)(?:\:(\d*))?/
With optional protocol and port:
/(?:(\w*)\:\/\/)?(?:(?:([\w\-]*)(?:\.))?([\w\-]*))\.(\w*)(?:\:(\d*))?/
URLs with path
Usually you'll want these to match specific protocols, therefore you can set them. In this case we'll be looking for http(s) and ftp(s). Otherwise you could also use the wildcard as in the general domain example.
/(https?|ftps?):\/\/([\w_-]+(?:(?:\.[\w_-]+)+))([\w.,@?^=%&:\/~+#-]*[\w@?^=%&\/~+#-])/
With optional protocol:
/(?:(https?|ftps?):\/\/)?([\w_-]+(?:(?:\.[\w_-]+)+))([\w.,@?^=%&:\/~+#-]*[\w@?^=%&\/~+#-])/
File paths
File path with filename + extension
/((\/|\\|\/\/|https?:\\\\|https?:\/\/)[a-z0-9 _@\-^!#$%&+={}.\/\\\[\]]+)+\.[a-z]+$/
File path with optional filename + extension
/^(.+)/([^/]+)$/
Filename with extension
/^[\w,\s-]+\.[A-Za-z]{3}$/
Date
YYYY/MM/DD
/([12]\d{3}-(0[1-9]|1[0-2])-(0[1-9]|[12]\d|3[01]))/
DD/MM/YYYY, DD-MM-YYYY, DD.MM.YYYY
/^(?:(?:31(\/|-|\.)(?:0?[13578]|1[02]))\1|(?:(?:29|30)(\/|-|\.)(?:0?[1,3-9]|1[0-2])\2))(?:(?:1[6-9]|[2-9]\d)?\d{2})$|^(?:29(\/|-|\.)0?2\3(?:(?:(?:1[6-9]|[2-9]\d)?(?:0[48]|[2468][048]|[13579][26])|(?:(?:16|[2468][048]|[3579][26])00))))$|^(?:0?[1-9]|1\d|2[0-8])(\/|-|\.)(?:(?:0?[1-9])|(?:1[0-2]))\4(?:(?:1[6-9]|[2-9]\d)?\d{2})$/
Time
HH:MM 12-hour
/^(0?[1-9]|1[0-2]):[0-5][0-9]$/
With meridiems (am/pm)
/((1[0-2]|0?[1-9]):([0-5][0-9]) ?([AaPp][Mm]))/
HH:MM 24-hour
/^([0-9]|0[0-9]|1[0-9]|2[0-3]):[0-5][0-9]$/
With required leading '0'
/^(0[0-9]|1[0-9]|2[0-3]):[0-5][0-9]$/
With Seconds
/(?:[01]\d|2[0123]):(?:[012345]\d):(?:[012345]\d)/
Other
username:password
/[0-9A-Za-z.]+:[0-z]+/
Single .env file line
/(?:^|^)\s*(?:export\s+)?([\w.-]+)(?:\s*=\s*?|:\s+?)(\s*'(?:\\'|[^'])*'|\s*\"(?:\\\"|[^\"])*\"|\s*`(?:\\`|[^`])*`|[^#\r\n]+)?\s*(?:#.*)?(?:$|$)/gm
International phone number incl. european notation
/[+]\d{1,2}|(00)\d{2,4}|\d{2})( [(]\d{1,3}[)])?[-. \/]?\d{2,6}?[-. \/]?\d{2,15}([-. \/]{1,3}\d{1,5}){0,4}/
Word duplicates
/(\b\w+\b)(?=.*\b\1\b)/ig
Social Security Number
/^((?!219-09-9999|078-05-1120)(?!666|000|9\d{2})\d{3}-(?!00)\d{2}-(?!0{4})\d{4})|((?!219 09 9999|078 05 1120)(?!666|000|9\d{2})\d{3} (?!00)\d{2} (?!0{4})\d{4})|((?!219099999|078051120)(?!666|000|9\d{2})\d{3}(?!00)\d{2}(?!0{4})\d{4})$/
Html tags with optional attributes
/<\/?[\w\s]*>|<.+[\W]>/